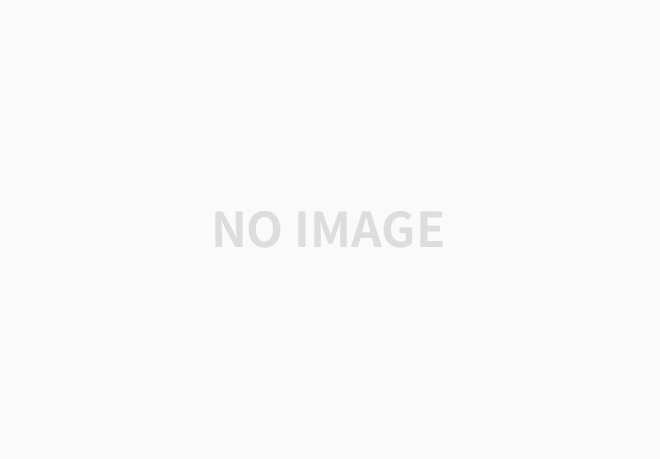
1. JavaScript Engine
Call Stack(호출 스택) : 코드들이 짜여진 순서대로 한 줄 한 줄 들어가서 실행되는 공간(single threaded : 한 번에 코드 한번 수행 가능)
Heap : 변수, 함수 등이 할당되는 공간
2. Web APIs
setTimeout과 같은 비동기 함수들(APIs)이 정의되어 있는 영역
비동기 함수들이 Call Stack에 들어오면 Web API 영역으로 이동된다고 생각하자.
이러한 비동기 함수들은 작업이 완료되면 callback 함수를 callback queue에 추가한다.
3. Callback Queue
비동기 함수 작업이 완료된 후, 실행이 되기 위해 다시 Call Stack에 들어가기 전에 대기하는 영역.
해당 함수가 어떤 형태이냐에 따라서 대기 순서가 바뀌는데 순서는 아래와 같다.
1. Microtask Queue(Job Queue) : Promise 함수
2. Animation Frames
3. Task Queue(Event Queue) : setTimeout 함수
console.log(1);
setTimeout(() => console.log("setTimeout"));
Promise.resolve() //
.then(() => console.log("Promise"));
console.log(2);
// 결과값
// 1
// 2
// "Promise"
// "setTimeout"
4. Event Loop
call stack에 작업중인 코드가 있는지 없는지 확인하는 과정.
call stack에 작업중인 코드가 없다면, callback queue에 대기하고 있던 작업들(콜백 함수)을 꺼내와서 call stack에 넘겨주는 역할을 한다.
5. JavaScript 내부 동작 원리 간단 정리
JavaScript는 기본적으로 웹 페이지를 작동시키기 위한 프로그래밍 언어이다. 웹페이지는 거의 즉각적이어야 한다.
JavaScript는 동기적으로 작동한다. 한 줄 한 줄(call stack).
그런데 즉각적으로 수행되지 못하는 코드들이 있다.(setTimeout, promise 등등)
그래서 JavaScript는 시간이 오래 걸리는 작업들을 Web APIs에게 넘겨버렸다.
이러한 코드들은 작업이 완료되면 콜백함수를 callback queue에 추가한다.
callback queue에 들어온 콜백함수들이 call stack에 들어가서 실행되기 위해서는 call stack에서 작업 중인 코드가 있는지 없는지 확인이 필요하다.
이러한 확인 절차를 거쳐 call stack에 아무것도 없을때 콜백 함수를 call stack에 추가해주는 것이 바로 event loop다.
참고 자료
'Programming > JavaScript' 카테고리의 다른 글
[JavaScript]동기와 비동기 개념 잡기 (0) | 2021.12.11 |
---|---|
[JavaScript]sessionStorage 간단 사용 (0) | 2021.11.25 |
[Javascript][Lecture][드림코딩]자바스크립트 기초 강의(ES5+)(13/13) (0) | 2021.11.24 |
[Javascript][Lecture][드림코딩]자바스크립트 기초 강의(ES5+)(12/13) (0) | 2021.11.24 |
[Javascript][Lecture][드림코딩]자바스크립트 기초 강의(ES5+)(11/13) (0) | 2021.11.10 |